Understanding HTTP Redirects: 301 vs. 302
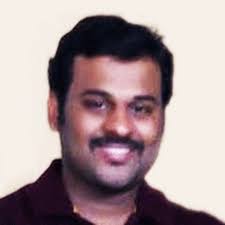
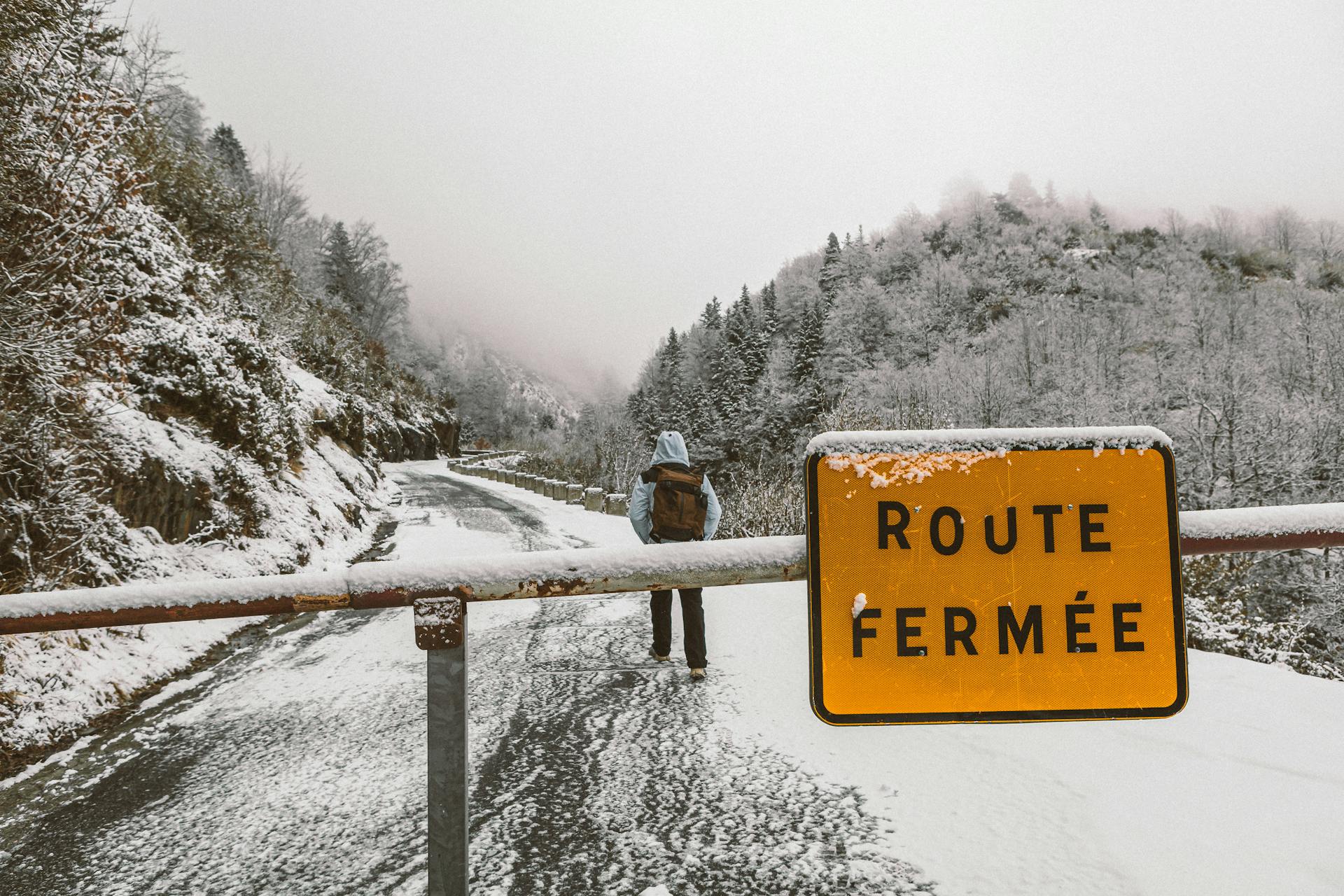
What Are 301 and 302 Redirects?
301 Redirect (Moved Permanently) : A 301 redirect tells browsers and search engines that a webpage has permanently moved to a new location. Search engines update their index to reflect the new URL and transfer the old URL's SEO value to the new one. Browsers cache the redirect, so future visits automatically go to the new URL.
302 Redirect (Found / Temporary) : A 302 redirect indicates a temporary move. Search engines keep the original URL indexed, assuming it'll return. Browsers don't cache the redirect as aggressively, checking the server for updates.
Using 301 and 302 Redirects in Node.js with Express
const express = require('express');
const app = express();
// 301 Redirect
app.get('/old-page-permanent', (req, res) => {
res.redirect(301, '/new-page');
});
// 302 Redirect
app.get('/old-page-temporary', (req, res) => {
res.redirect(302, '/new-page');
});
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
// Visiting /old-page-permanent results in a permanent redirect to /new-page.
// Visiting /old-page-temporary results in a temporary redirect to /new-page.
When to Use Which?
301 Redirects: For permanent moves, like restructuring a site or changing domains. Transfers SEO value to the new URL. If you move your blog from /blog-old to /blog-new: Use a 301 redirect for a permanent move. Search engines will index /blog-new, and users will land there seamlessly.
302 Redirects: For temporary changes, like maintenance or A/B testing. Keeps the original URL's SEO value. Use a 302 redirect for temporary changes, like a redesign. The original URL stays indexed, and you can revert traffic later.