Getting Started with Lynx: A Step-by-Step Guide
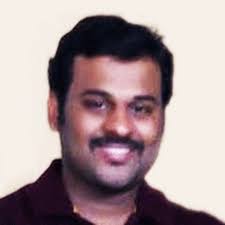
Lynx is an emerging mobile development framework that bridges the gap between native performance and JavaScript flexibility. Whether you're a frontend developer looking to build mobile apps or an experienced native developer exploring new tools, Lynx offers a lightweight and efficient way to create high-performance applications.
In this guide, we’ll walk through setting up a Lynx project from scratch, running it on a real device, and integrating native features like the camera and geolocation. By the end, you'll have a working mobile app powered by Lynx. Let's dive in! 🚀
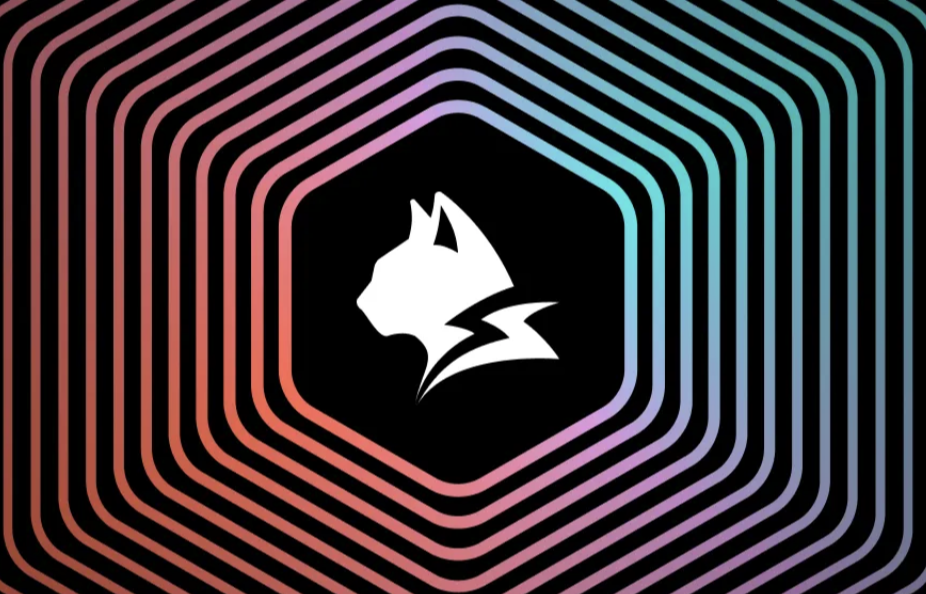
Step 1: Install Lynx CLI
Ensure you have Node.js installed, then install Lynx's command-line tool:
npm install -g lynx-cli
Step 2: Create a New Lynx Project
Generate a new project and navigate into it:
lynx init my-lynx-app
cd my-lynx-app
Step 3: Run the App on a Device or Emulator
To test on an Android device/emulator:
lynx run android
For iOS (Mac users with Xcode installed):
lynx run ios
Step 4: Install Lynx Camera & Geolocation Modules
Lynx provides native modules to access device capabilities. Install the camera and geolocation modules:
lynx install lynx-camera lynx-geolocation
Using Lynx to Capture an Image and Get User Location
Now, let's implement an example where we:
- Open the camera and capture an image.
- Retrieve the user's current location .
- Display both on the screen.
Creating a Camera Button
We first create a button to open the device camera:
import { createElement } from "lynx-ui";
import { NativeBridge } from "lynx-bridge";
const openCameraButton = createElement("Button", {
text: "Capture Photo",
onClick: async () => {
try {
// Call the native camera API
const imageUri = await NativeBridge.call("Camera.open");
console.log("Captured Image URI:", imageUri);
// Display the captured image
displayCapturedImage(imageUri);
} catch (error) {
console.error("Camera access error:", error);
}
},
});
lynxRoot.appendChild(openCameraButton);
Retrieving User Location
Now, let's fetch the user's current GPS location and display it.
const getLocationButton = createElement("Button", {
text: "Get My Location",
onClick: async () => {
try {
const location = await NativeBridge.call("Geolocation.getCurrentPosition");
console.log("User Location:", location);
// Display location coordinates
displayLocation(location);
} catch (error) {
console.error("Error fetching location:", error);
}
},
});
lynxRoot.appendChild(getLocationButton);
Displaying the Captured Image and Location
Once the user captures an image and retrieves their location, we display both in the UI.
function displayCapturedImage(imageUri) {
const imageView = createElement("ImageView", {
src: imageUri,
width: "100%",
height: "auto",
});
lynxRoot.appendChild(imageView);
}
function displayLocation(location) {
const locationText = createElement("Text", {
fontSize: 16,
});
lynxRoot.appendChild(locationText);
}
Running the App
To test the app on a real device, build and deploy it. This will install and launch the app on your connected test device.
lynx build
lynx run android # or lynx run ios
Wrapping Up: Why Lynx is Worth Exploring
Lynx is redefining mobile development with its innovative dual-engine architecture, native rendering path, and modular layout system. By combining high performance with JavaScript flexibility, it offers a compelling solution for developers looking to build seamless, efficient mobile apps.
Now that you’ve set up your first Lynx project, it’s time to dive deeper. But what makes Lynx so fast and efficient? How do its design choices compare to other frameworks?
Next : Understanding Lynx's Design Choices for High-Performance Mobile Development
Discover the core principles behind Lynx’s high-performance architecture in my next article 👇. See how its unique approach could change the way you build mobile apps! 🚀